Top 10 JavaScript Tips and Tricks for 2023: Boost Your Coding Skills
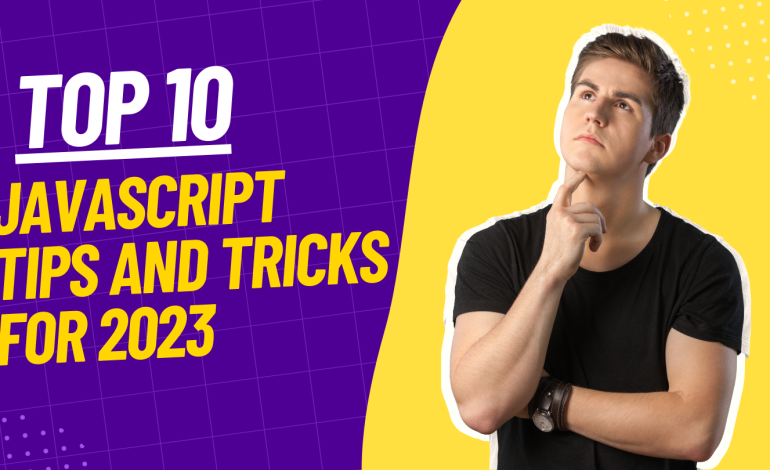
JavaScript continues to be one of the most popular programming languages in 2023, and mastering it is essential for web developers. In this article, we’ll explore JavaScript tips and tricks for 2023 to help you improve your JavaScript skills, write more efficient code, and keep up with the latest trends. These practical examples will help you understand advanced concepts more easily and enable you to implement them in your projects.
Use modern JavaScript syntax (ES6+)
Modern JavaScript syntax, introduced with ECMAScript 2015 (ES6) and later versions, brings many improvements that make your code more concise and easier to read. Some key features include:
- Arrow functions: Arrow functions provide a shorter syntax for writing function expressions and automatically bind
this
to the surrounding scope. Example:
const numbers = [1, 2, 3];
const doubled = numbers.map(number => number * 2);
- Template literals: Template literals allow you to embed expressions inside string literals, using
${expression}
. Example:
const name = 'John';
const greeting = `Hello, ${name}!`;
- Destructuring assignments: Destructuring makes it easy to extract values from arrays or properties from objects into distinct variables. Example:
const [first, , third] = [1, 2, 3];
console.log(first, third); // Output: 1 3
By using modern JavaScript syntax, you’ll write cleaner, more maintainable code and take advantage of the latest language features.
Master the spread and rest operators
The spread and rest operators, represented by ...
, are versatile tools for working with arrays and objects.
- Spread operator: The spread operator allows you to expand elements of an iterable (like an array) in places where multiple arguments or elements are expected. Example:
const array1 = [1, 2, 3];
const array2 = [4, 5, 6];
const combinedArray = [...array1, ...array2];
console.log(combinedArray); // Output: [1, 2, 3, 4, 5, 6]
Rest operator: The rest operator is used in function arguments to collect the remaining arguments into a single array. Example:
function sum(...numbers) {
return numbers.reduce((total, num) => total + num, 0);
}
console.log(sum(1, 2, 3, 4, 5)); // Output: 15
Understanding and utilizing the spread and rest operators can greatly simplify your code and make it more readable.
Understand and use Promises and async/await
Promises and async/await are powerful tools for handling asynchronous operations in JavaScript.
- Promises: A Promise represents the eventual result of an asynchronous operation. It can be in one of three states: pending, fulfilled, or rejected. Promises allow you to chain multiple asynchronous operations and handle errors more easily. Example:
fetch('https://api.example.com/data')
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error(error));
- Async/await: Async/await is a more readable way to work with Promises. A
async
function always returns a Promise, and theawait
keyword can be used inside anasync
function to pause the execution until the Promise is resolved. Example:
async function fetchData() {
try {
const response = await fetch('https://api.example.com/data');
const data = await response.json();
console.log(data);
} catch (error) {
console.error(error);
}
}
fetchData();
Using async/await can make your asynchronous code more readable and easier to maintain.
Use the optional chaining operator
The optional chaining operator ?.
is a useful feature introduced in ES2020 that simplifies accessing properties of objects that might be undefined or null. Instead of checking for the existence of each property in a nested object, you can use the optional chaining operator. Example:
const user = {
name: 'John',
address: {
street: 'Main St',
city: 'New York',
},
};
const city = user?.address?.city;
console.log(city); // Output: New York
Using optional chaining can help you avoid errors caused by attempting to access properties on undefined or null objects.
Use nullish coalescing operator
The nullish coalescing operator ??
is another useful feature introduced in ES2020. It returns the right-hand operand when the left-hand operand is null or undefined; otherwise, it returns the left-hand operand. This is useful for providing default values. Example:
const user = {
name: 'John',
age: 0,
};
const age = user.age ?? 18;
console.log(age); // Output: 0
By using the nullish coalescing operator, you can simplify your code and provide default values more effectively.
Master JavaScript array methods
JavaScript provides a plethora of array methods that can help you manipulate and iterate over arrays more easily. Some popular array methods include:
map()
: creates a new array with the results of calling a provided function on every element in the calling array.filter()
: creates a new array with all elements that pass the test implemented by the provided function.reduce()
: applies a function against an accumulator and each element in the array (from left to right) to reduce it to a single value.
Mastering these array methods will help you write more efficient and readable code.
Learn to debug JavaScript effectively
Effective debugging is a crucial skill for any developer. Some useful debugging techniques include:
- Using
console.log()
,console.error()
, andconsole.table()
to print messages and data to the console. - Leveraging browser developer tools, such as breakpoints, the debugger, and performance profiling.
- Writing unit tests to catch bugs early and ensure the correctness of your code.
By mastering debugging techniques, you’ll be able to quickly identify and fix issues in your code.
Stay up-to-date with the latest JavaScript features and best practices
JavaScript is an evolving language, with new features and best practices being introduced regularly. Staying up-to-date with these changes will help you write better code and stay competitive in the job market. Some ways to stay informed include:
- Following JavaScript blogs and newsletters.
- Participating in online communities and attending meetups or conferences.
- Taking online courses or workshops to learn new skills.
Summary
By incorporating these tips and tricks into your JavaScript development, you’ll be able to write more efficient, maintainable, and future-proof code. Keep practicing and refining your skills to become an even better JavaScript developer in 2023 and beyond.