Fetching Data in Next.js: How to Get Started
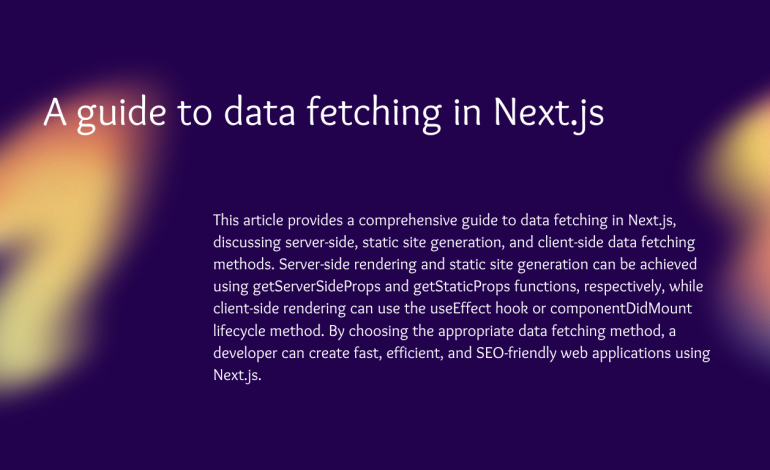
Data fetching is a crucial aspect of web application development. In Next.js, there are several ways to fetch data in your application, and it can be challenging to determine which one is the best fit for your needs. In this article, we’ll cover everything you need to know about data fetching in Next.js.
Server-Side Rendering
One of the primary benefits of Next.js is its support for server-side rendering (SSR). SSR allows you to render your application on the server, which can improve its performance and SEO. In Next.js, you can fetch data during the server-side rendering process using the getServerSideProps
function.
javascriptCopy codefunction MyComponent({ data }) {
// Render your component using the data
}
export async function getServerSideProps(context) {
// Fetch data from an external API
const response = await fetch('https://myapi.com/data');
const data = await response.json();
return {
props: {
data
}
}
}
In this example, we define an async function called getServerSideProps
that fetches data from an external API and passes it as a prop to our component. The getServerSideProps
function is executed on the server during the rendering process, so the data is fetched before the component is rendered.
Static Site Generation
Next.js also supports static site generation (SSG), which can further improve the performance and SEO of your application. In Next.js, you can fetch data during the SSG process using the getStaticProps
function.
javascriptCopy codefunction MyComponent({ data }) {
// Render your component using the data
}
export async function getStaticProps() {
// Fetch data from an external API
const response = await fetch('https://myapi.com/data');
const data = await response.json();
return {
props: {
data
}
}
}
In this example, we define an async function called getStaticProps
that fetches data from an external API and passes it as a prop to our component. The getStaticProps
function is executed during the build process, so the data is fetched before the application is deployed.
Client-Side Rendering
If you need to fetch data after the initial render, you can use client-side rendering (CSR) in Next.js. You can use the useEffect
hook or the componentDidMount
lifecycle method to fetch data on the client-side.
javascriptCopy codefunction MyComponent() {
const [data, setData] = useState(null);
useEffect(() => {
async function fetchData() {
// Fetch data from an external API
const response = await fetch('https://myapi.com/data');
const data = await response.json();
setData(data)
}
fetchData();
}, []);
// Render your component using the data
}
In this example, we use the useEffect
hook to fetch data from an external API after the component has mounted. The fetched data is stored in state using the useState
hook, and then rendered by the component.
Conclusion
Next.js provides several options for fetching data depending on your application’s needs. Whether you need to fetch data during server-side rendering, static site generation, or client-side rendering, the framework has you covered. By using the appropriate data fetching method, you can create fast, efficient and SEO-friendly web applications.
1 Comment
[…] Async/await is a more readable way to work with Promises. A async function always returns a Promise, and the await keyword can be used inside an async […]