Performance Optimization in Next.js: Tips and Techniques for Fast Web Applications
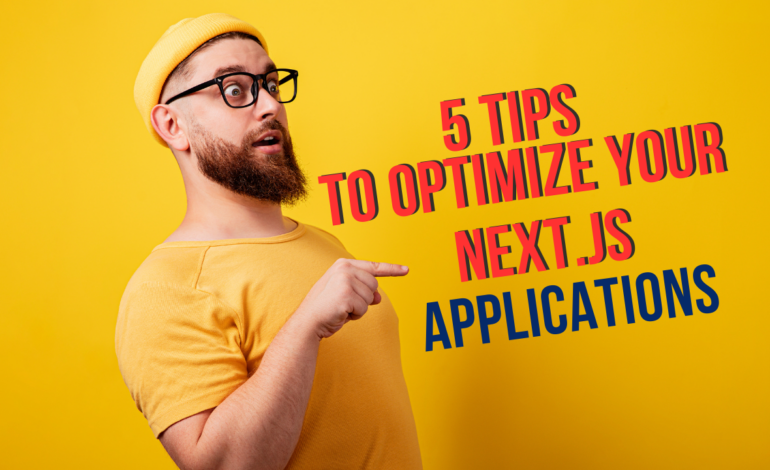
Next.js is a popular React framework that offers many features to help you build fast and scalable web apps. However, as your app grows and becomes more complex, you might encounter some performance issues that can affect your user experience and SEO ranking. In this blog post, We will discover some tips and techniques about Next.js performance optimization to make faster web applications.
#performance-optimization #next.js #react #ux #seo
1. Use Dynamic Imports for Lazy Loading
One of the main benefits of Next.js is that it automatically splits your code into smaller chunks based on your pages and routes. This means that your app only loads the code that is necessary for each page, reducing the initial loading time and improving the user experience. However, sometimes you might have some components or modules that are not essential and used in every page load, but still, take up a lot of space in your bundle. For example, you might have a component that renders a chart or a map, but it is only used when a user scrolls down and reaches it. In this case, you can use dynamic imports to load these components or modules only when they are needed. Dynamic imports are a feature of JavaScript that allows you to import a module or a component asynchronously, using a function call. Next.js provides a built-in component called dynamic that makes it easy to use dynamic imports in your app. For example, if you have a component called Chart that is only used on one page of your app, you can import it dynamically like this:
import dynamic from 'next/dynamic'
const Chart = dynamic(() => import('../components/Chart'))
By using dynamic imports, you can reduce the size of your bundle and improve the performance of your app.
2. Optimize Images
Images are often one of the biggest contributors to the size and loading time of your web pages. If you use large or unoptimized images in your app, they can significantly slow down your app and affect your user experience and SEO ranking. Fortunately, Next.js provides a powerful image optimization component that can help you optimize your images automatically. You can use this Image component instead of the standard HTML <img /> tag in your app, and provide some props to customize its behavior. For example, you can use the width and height props to specify the dimensions of your image, the priority prop to adjust if the image is your Largest Content Paint of your page, and the fill prop to define your image should fill its container. Here is an example of using the Image component in your app:
import Image from 'next/image'
import Logo from '/logo.png'
<Image
src={Logo}
alt="Logo"
width={200}
height={200}
priority
/>
By using the Image component from Next.js, you can optimize your images and improve the performance of your app.
3. Cache Frequently Used Content
Another way to improve the performance of your Next.js app is to cache frequently used content on the server or the client side. Caching means storing some data or content in a temporary storage location so that it can be retrieved faster when needed again. Caching can reduce the number of requests to your server or external APIs, save bandwidth and resources, and improve the user experience and SEO ranking of your app. There are different ways to implement caching in your Next.js app, depending on your needs and preferences. For example, you can use server-side caching techniques such as stale-while-revalidate or cache-control headers to cache some data or content on your server and serve it faster to your clients. You can also use client-side caching techniques such as localStorage or service workers to cache some data or content on your browser and access it offline or when there is no network connection. Here is an example of using localStorage to cache some data on your browser:
const data = localStorage.getItem('cachedData')
if (data ) {
const parsedData = JSON.parse(data)
...
} else {
fetch('/api/data')
.then(response => response.json())
.then(data => localStorage.setItem('cachedData',data))
4. Use Incremental Static Regeneration
Next.js supports three types of rendering: static generation, server-side rendering, and hybrid rendering. Static generation is the preferred method for most pages, as it allows you to pre-render your pages at build time and serve them as static files. This can improve the performance and security of your app, as well as enable features like CDN caching and offline support.
However, sometimes you may need to update your static pages with fresh data from an API or a database. In this case, you can use incremental static regeneration, which allows you to re-generate your static pages in the background after a request. This way, you can always serve the latest version of your pages without sacrificing performance or user experience.
You can use incremental static regeneration by specifying a revalidate property in the getStaticProps function. This property determines how often (in seconds) a page should be re-generated. For example:
export async function getStaticProps() {
const data = await fetch('https://api.example.com/data')
return {
props: {
data
},
// Re-generate the page every hour
revalidate: 3600
}
}
Using incremental static regeneration can help you keep your static pages up-to-date without compromising on performance.
5. Analyze and Optimize Your Bundle Size
Finally, one of the best ways to optimize your Next.js app performance is to analyze and optimize your bundle size. The bundle size is the amount of JavaScript code that is sent to the browser when a user visits your app. The smaller the bundle size, the faster your app loads and runs.
Next.js provides a plugin for analyzing your bundle size called @next/bundle-analyzer. This plugin generates an interactive treemap visualization of your bundle size, which can help you identify and eliminate unnecessary or redundant code from your code bundles. If you were interested in how to use this plugin I suggest checking this amazing Youtuve video from Patreon here:
Conclusion
In conclusion, optimizing the performance of Next.js web applications is crucial to deliver a fast and seamless user experience. By adopting the techniques and tips outlined in this article, you can achieve faster load times and better performance for your web applications. Using strategies like caching, optimizing images, and implementing server-side rendering/incremental static regeneration can help improve overall performance and SEO ranking.
That’s it for this article and if you are interested in learning more about Next.js don’t forget to check out my previous articles on Deploying Next.js Applications and Dynamic Rendering in Next.js.