Master Asynchronous JavaScript in 2023: A Comprehensive Guide
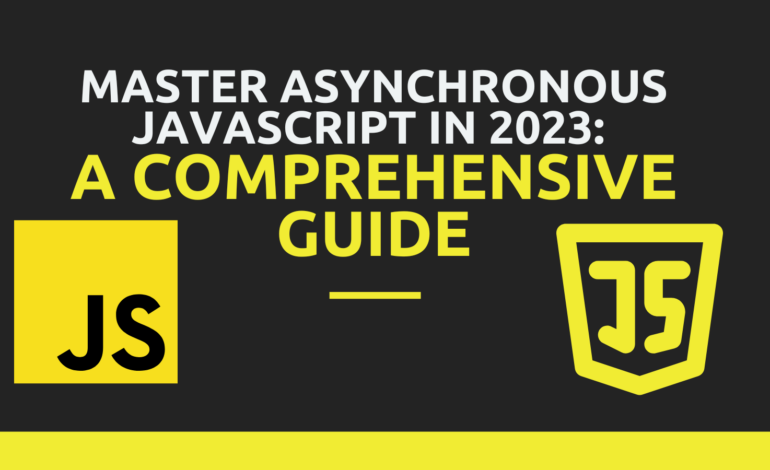
Asynchronous JavaScript in 2023 has become a critical skill for frontend developers in recent years, as it allows us to build highly responsive and performant web applications. In this article, we will cover everything you need to know to become a master of asynchronous JavaScript in 2023.
Part 1: Callbacks
Callbacks are the oldest and most fundamental way of handling asynchronous operations in JavaScript. A callback is simply a function that is passed as an argument to another function, and is executed when an operation completes. Here is an example:
function fetchData(url, callback) {
let xhr = new XMLHttpRequest();
xhr.onreadystatechange = function() {
if (xhr.readyState === 4 && xhr.status === 200) {
callback(xhr.responseText);
}
};
xhr.open('GET', url);
xhr.send();
}
fetchData('https://jsonplaceholder.typicode.com/todos/1', function(data) {
console.log(data);
});
In this example, we define a fetchData()
function that takes a URL and a callback function as parameters. We create an XMLHttpRequest
object and set its onreadystatechange
property to a function that checks if the response is ready and successful, and calls the callback function with the response text if so.
We then call the fetchData()
function with a URL and an anonymous callback function that logs the data to the console.
Callbacks can quickly become messy and hard to read when nested deeply, leading to what is commonly known as “callback hell”. To avoid this, it’s best to use named functions and modularize your code as much as possible.
Part 2: Promises
Promises provide a more structured way to handle asynchronous operations in JavaScript. A promise is an object that represents the eventual completion (or failure) of an asynchronous operation. Promises can be chained and handled using then()
and catch()
methods. Here is an example:
function fetchData(url) {
return new Promise((resolve, reject) => {
let xhr = new XMLHttpRequest();
xhr.onreadystatechange = function() {
if (xhr.readyState === 4) {
if (xhr.status === 200) {
resolve(xhr.responseText);
} else {
reject(new Error('Network response was not ok'));
}
}
};
xhr.open('GET', url);
xhr.send();
});
}
fetchData('https://jsonplaceholder.typicode.com/todos/1')
.then(data => {
console.log(data);
})
.catch(error => {
console.error(error);
});
In this example, we define a fetchData()
function that returns a new promise. We create an XMLHttpRequest
object and set its onreadystatechange
property to a function that resolves the promise with the response text if the request is successful, or rejects it with an error if not.
We then call the fetchData()
function with a URL, chain then()
and catch()
methods to handle the promise. If the promise is resolved, we log the data to the console. If the promise is rejected, we log the error to the console.
Promises provide a more structured and readable way of handling asynchronous operations than callbacks, and can be used with modern syntax such as async/await
.
Part 3: Async/Await Syntax
Async/await syntax is a more modern way of handling asynchronous operations in JavaScript, which provides a cleaner and more concise way of writing asynchronous code. Here is an example:
async function fetchData(url) {
let response = await fetch(url);
if (!response.ok) {
throw new Error('Network response was not ok');
}
let data = await response.json();
console.log(data);
}
fetchData('https://jsonplaceholder.typicode.com/todos/1')
.catch(error => {
console.error(error);
});
In this example, we define an async
function called fetchData()
that uses the await
keyword to wait for the response of a fetch()
call. If the response is not ok
, we throw an error. Otherwise, we parse the response as JSON and log it to the console.
We then call the fetchData()
function with a URL and catch any errors that may be thrown.
Async/await syntax provides a more readable and less error-prone way of handling asynchronous operations than callbacks and promises, and can be used with modern features like destructuring and object shorthand notation.
Part 4: Reactive Programming with RxJS
Reactive programming with RxJS is a powerful way of handling asynchronous data streams in JavaScript. RxJS uses observables to represent streams of data, and provides a wide range of operators to transform and manipulate those streams. Here is an example:
const { from } = rxjs;
const { filter, map } = rxjs.operators;
from(fetch('https://jsonplaceholder.typicode.com/todos/1'))
.pipe(
filter(response => response.ok),
map(response => response.json())
)
.subscribe(data => {
console.log(data);
}, error => {
console.error(error);
});
In this example, we use the from()
method from RxJS to create an observable from a fetch()
call. We then use the filter()
and map()
operators to transform the observable, filtering out non-OK responses and parsing the response as JSON.
We then subscribe to the observable to log the data to the console or catch any errors that may be thrown.
Reactive programming with RxJS provides a powerful way of handling asynchronous data streams that is both readable and maintainable, especially when dealing with large and complex data sets.
Conclusion
In this article, we have covered the basics of asynchronous programming in JavaScript, including callbacks, promises, async/await syntax, and reactive programming with RxJS. By mastering these techniques, you will be well-equipped to build responsive and performant web applications in 2023 and beyond. Keep practicing and experimenting with different scenarios, and stay up to date with the latest developments in JavaScript and web development.