Next.js for Beginners: A Comprehensive Introduction to the Next.js Framework
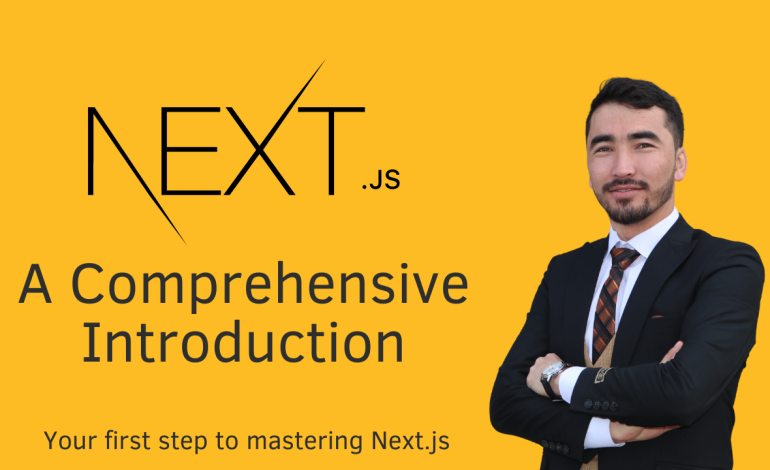
If you are looking for a way to create fast and modern web applications with React, you might have heard of Next.js. Next.js is a framework that helps you build production-ready React applications with minimal configuration and great developer experience. In this blog post, I will give you a comprehensive introduction to Next.js and show you how to get started with it.
What You Will Learn in This Article?
- What is Next.js and Why Use it?
- How to Get Started with Next.js?
- Understanding the File Structure of a Next.js Application
- How to Create Your First Page in Next.js?
- Routing and Navigation in Next.js
- Data Fetching and API Routes
- Deploying Your Next.js App
What is Next.js and Why Use it?
Next.js is a framework that adds some features and enhancements to React, such as:
– Server Side Rendering (SSR): Next.js can render your pages on the server and send them to the browser as HTML, which improves the performance and SEO of your application.
– Static Site Generation (SSG): Next.js can also pre-render your pages at build time and serve them as static files, which makes your site even faster and more secure.
– Incremental Static Regeneration (ISR) is a feature in Next.js that allows you to update static pages without having to rebuild the entire site. This means that you can update your website’s content in real-time, without any downtime or interruption to your users.
– File based routing: Next.js automatically creates routes based on the files in your pages directory, so you don’t have to write any routing logic yourself like how you do in React applications.
– API routes: Next.js lets you create API endpoints in your pages directory, which are serverless functions that can handle requests and responses without the need for any separate server.
– Built-in CSS and Sass support: Next.js allows you to import CSS and Sass files in your components, and handles the bundling and minification for you.
– Image optimization: Next.js provides an Image component that automatically optimizes your images for different screen sizes and formats.
– And much more: Next.js also comes with features like code splitting, hot reloading, TypeScript support, custom hooks, plugins, and more.
How to Get Started With Next.js?
To get started with Next.js, you need to have Node.js and npm or any supported package managers installed on your machine. Then, you can use the create-next-app command to create a new Next.js project:
npx create-next-app hello-nextjs
This will guide you through some steps of choosing Yes/No options to set you with advanced setups like setting TypeScript, activating the experimental app directory, and setting TailwindCSS but for this intro blog, you create a project called hello-nextjs with the most basic configuration. Then cd into the project directory and run npm run dev to start the development server:
cd hello-nextjs
npm run dev
You can now open http://localhost:3000 in your browser and see a welcome page. You can also edit the files in the pages directory and see the changes reflected in the browser.
Understanding the File Structure of a Next.js App
One of the benefits of Next.js is that it has a simple and intuitive file structure that makes it easy to organize your code and components. Here, we will explore the main folders and files that you will encounter when working with Next.js.
The root folder of a Next.js project contains some configuration files and folders that are essential for the framework to work. These include:
package.json file
This file contains the dependencies and scripts for your project. You can use `npm` or `yarn` to install and run them.
next.config.js file
This file allows you to customize the behavior and features of Next.js, such as adding environment variables, customizing webpack, enabling experimental features support, etc. You can learn more about the options available in this file from the official documentation.
pages directory
This folder contains all the pages of your application. Each file in this folder corresponds to a route in your app. For example, `index.js` is mapped to the `/` route, and `about.js` is mapped to the `/about` route. You can also create dynamic routes using brackets, such as `[id].js`, which will match any value after the `/` in the URL. Next.js will automatically handle the rendering and hydration of these pages on the server or the client, depending on your configuration.
public directory
This folder contains any static assets that you want to serve with your app, such as images, fonts, icons, etc. You can access these assets by using the root URL (`/`) as the base path. For example, if you have an image called `logo.png` in the `public` folder, you can use `<img src=”/logo.png” />` in your JSX code to display it.
styles directory
This folder contains any global CSS styles that you want to apply to your Next.js app. You can use any CSS-in-JS library or preprocessor with Next.js, but by default, it supports importing CSS modules and Sass files. To use CSS modules, you need to name your files with the `.module.css` extension, and then import them in your components like this: `import styles from ‘./styles.module.css’`. To use Sass, you need to install `sass` as a dependency and name your files with the `.scss` or `.sass` extension.
These are the main folders and files that you will see in a Next.js project, but you can also create your own folders and files to organize your code according to your needs. For example, you can create a `components` folder to store your reusable React components, or a `lib` folder to store your utility functions and API calls. The only rule is that you should not create any files or folders with names that conflict with the ones used by Next.js, such as `_app.js`, `_document.js`, or `api`.
How to Create Pages in Next.js?
As mentioned before, Next.js uses file-based routing, which means that every file in the pages directory corresponds to a route in your app. For example, if you create a file called about.jsx in the pages directory, it will be accessible at http://localhost:3000/about.
To create a page in Next.js, you need to export a React component from that file. For example, here is a simple About page:
// pages/about.jsx
import React from 'react'
export default function About() {
return (
<div>
<h1>About</h1>
<p>This is a Next.js app.</p>
</div>
)
}
You can also use dynamic routes to create pages based on parameters. For example, if you want to create a page for each blog post, you can use brackets to indicate a dynamic segment in the file name:
// pages/posts/[postId].jsx
import React from 'react'
export default function Post({ post }) {
return (
<div>
<h1>{post.title}</h1>
<p>{post.content}</p>
</div>
)
}
// This function runs on the server and fetches the data for the page
export async function getServerSideProps(context) {
// Get the postId from the query
const { postId } = context.query
// Fetch the post from an API
const res = await fetch(`https://jsonplaceholder.typicode.com/posts/${postId}`)
const post = await res.json()
// Return the post as props
return {
props: {
post,
},
}
}
This will create a page for each post at http://localhost:3000/posts/[postId], where [postId] is the id of the post. You can also use getStaticProps instead of getServerSideProps if you want to pre-render the page at build time.
Routing and Navigation in Next.js
Routing is the process of matching a URL to a page component. Next.js has a file-based routing system, which means that you can create pages by simply adding files to the pages directory. For example, if you create a file called about.js in the pages directory, it will be accessible at /about.
Navigation is the process of moving from one page to another. Next.js provides a Link component that allows you to create links to other pages in your app. For example, if you want to link to the About page from the index page, you can write something like this:
<Link href="/about">About</Link>
The Link component will automatically prefetch the page in the background, making the navigation faster and smoother.
Data Fetching and API Routes
One of the features that Next.js provides is the ability to create API routes that can handle requests from your frontend or external sources. API routes are essentially functions that run on the server and respond to HTTP requests. You can use them to perform any server-side logic, such as fetching data from a database, sending emails, or processing payments. API routes are also useful for creating RESTful APIs that can be consumed by other applications.
To create an API route in Next.js, you need to create a file under the `pages/api` directory with the `.js` extension. The name of the file will determine the URL of the API route. For example, if you create a file called `pages/api/hello-next.js`, you will have an API route at `/api/hello-next`.
The file should export a default function that receives two parameters: `req` and `res`. The `req` parameter is an instance of the Node.js http.IncomingMessage class, which represents the incoming request. The `res` parameter is an instance of the Node.js http.ServerResponse class, which represents the outgoing response.
The function should use the `res` parameter to send back a response to the client. You can use methods like `res.status()` to set the HTTP status code, `res.json()` to send a JSON object, or `res.send()` to send any other type of data.
For example, here is a simple API route that returns a JSON object with a greeting message:
// pages/api/hello-next.js
export default function handler(req, res) {
res.status(200).json({ message: 'Hello from Next.js api routes!' });
}
You can test this API route by visiting `http://localhost:3000/api/hello` in your browser or using a tool like Postman. You should see something like this:
{
“message”: “Hello from Next.js api routes!”
}
Using API Routes in Frontend Components
To use an API route in your frontend components, you can use the built-in fetch API or any other library that can make HTTP requests. You can use the `getStaticProps` or `getServerSideProps` functions to fetch data from your API routes at build time or request time, respectively. You can also use client-side rendering techniques like SWR or React Query to fetch data from your API routes on the browser.
For example, here is a simple component that fetches data from the `api/hello-next` route and displays it on the screen:
// pages/index-next.js
import { useEffect, useState } from 'react';
export default function Home() {
const [data, setData] = useState(null);
useEffect(() => {
// Fetch data from /api/hello-next
fetch('/api/hello-next')
.then((res) => res.json())
.then((data) => setData(data));
}, []);
return (
<div>
<h1>Home</h1>
{data ? (
<p>{data.message}</p>
) : (
<p>Loading…</p>
)}
</div>
);
}
You can run this component by visiting `http://localhost:3000/` in your browser. You should see something like this:
<h1>Home</h1>
<p>Hello from Next.js api rountes!</p>
Deploying Your Next.js App
To deploy your Next.js app, you’ll need two things: a hosting service and a domain name. There are many hosting services and domain name providers out there, but for this tutorial, we’re going to use Vercel. Vercel is the company behind Next.js and they offer a free and easy way to deploy your Next.js app with zero configuration. They also provide a custom domain name for your app, which you can change later if you want.
Here are the steps to deploy your Next.js app with Vercel:
- Create a Vercel account at https://vercel.com/signup. You can sign up with your email, GitHub, GitLab, or Bitbucket account.
- Install the Vercel CLI (command-line interface) on your computer by running `npm install -g vercel` in your terminal. This will allow you to interact with Vercel from your command line.
- Go to the directory where your Next.js app is located and run `vercel login` in your terminal. This will authenticate you with your Vercel account.
- Run `vercel` in your terminal. This will deploy your app to a temporary URL that looks something like https://hello-nextjs.vercel.app.
- Visit the URL in your browser and see your app live on the web!
That’s it! You’ve just deployed your Next.js app to the web with Vercel. You can now share your app with anyone and enjoy its fast performance and rich features.
Conclusion
In conclusion, Next.js is a powerful and popular framework for building complex web applications. With its built-in features and integration with React, Next.js makes it easy for beginners to build fast and optimized web applications. Its easy setup process, automatic routing, and static site generation capabilities make it flexible and efficient for a wide range of use cases. Overall, Next.js is an excellent choice for your next web development project. You can learn more about Next.js features from the official documentation. And if you are eager to learn more about Next.js read my preview article about Static Site Generations with Next.js, or if you are a fan of video-based tutorials like me I suggest you watch this awesome crash course from Traversy Media about Nextjs 13 and its new features and changes.
Thanks for reading and happy coding!
2 Comments
[…] If you want to know more about Next.js read my previews article about Next.js here: […]
[…] If you are interested to read more about Next.js check out my previews article “Next.js for Beginners: A Comprehensive Introduction to the Next.js Framework” […]