How to Master Tailwind CSS Plugin Development in Your Next.js Projects: A Comprehensive Guide
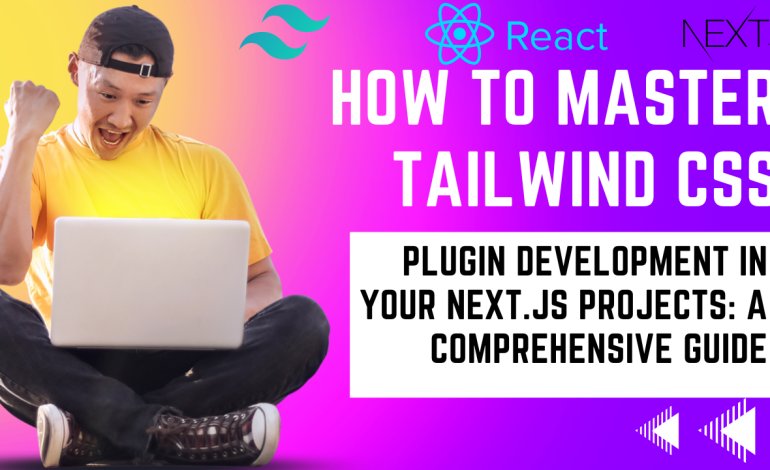
In this blog post, we will discuss the importance and advantages of creating your own Tailwind CSS plugins and demonstrate how to create a simple plugin in a Next.js project.
Introduction
Tailwind CSS is a popular utility-first CSS framework designed to make it easy to style modern applications. By utilizing Tailwind CSS plugins, you can extend the framework’s functionality and make your codebase more consistent and maintainable.
Why create your own Tailwind CSS plugins?
There are several reasons why you may want to create your own Tailwind CSS plugins:
- Consistency: Custom plugins allow you to define a set of styles that can be reused across your application. This ensures that your design remains consistent, making it easier to manage and maintain.
- Customization: Plugins enable you to create your own utility classes that are tailored to your project’s specific needs. This allows you to add custom functionality to Tailwind CSS without having to write repetitive, inline styles.
- Reusability: Once you have created a plugin, you can easily share it with other projects or even publish it for the community to use. This promotes reusability and helps you maintain a consistent design system across multiple projects.
- Efficiency: Writing custom plugins allows you to define complex styles and behaviors with simple utility classes. This can make your code more readable and easier to understand, increasing your team’s productivity and efficiency.
With these benefits in mind, let’s dive into creating a custom Tailwind CSS plugin in a Next.js project.
Step 1: Set up the Next.js project
First, let’s create a new Next.js project using the following command:
npx create-next-app customPlugin
//change directory
cd customPlugin
Step 2: Install Tailwind CSS
Now, let’s install Tailwind CSS and its dependencies:
npm install -D tailwindcss@latest postcss@latest autoprefixer@latest
Create a configuration file for Tailwind CSS:
npx tailwindcss init -p
This command will create a tailwind.config.js
and a postcss.config.js
file in your project directory.
Step 3: Configure Tailwind CSS
Open tailwind.config.js
and add the plugin configuration:
module.exports = {
purge: ['./pages/**/*.{js,ts,jsx,tsx}', './components/**/*.{js,ts,jsx,tsx}'],
darkMode: false,
theme: {
extend: {},
},
variants: {
extend: {},
},
plugins: [require('./plugins/loading-indicator')],
}
Step 4: Create the loading-indicator plugin
Create a new directory called plugins
:
mkdir plugins
//Create a new file called loading-indicator.js inside the plugins directory:
touch plugins/loading-indicator.js
Open the loading-indicator.js
file and add the following code:
const plugin = require('tailwindcss/plugin')
module.exports = plugin(({ addUtilities }) => {
const loadingUtilities = {
'.loading-indicator': {
display: 'inline-block',
border: '2px solid transparent',
borderTop: '2px solid currentColor',
borderRadius: '50%',
width: '24px',
height: '24px',
animation: 'spin 1s linear infinite',
},
}
addUtilities(loadingUtilities, ['responsive'])
})
This plugin creates a .loading-indicator
utility class that styles a loading spinner.
Step 5: Include Tailwind CSS in your project
Open the styles/globals.css
file and replace its content with the following:
@tailwind base;
@tailwind components;
@tailwind utilities;
@keyframes spin {
to {
transform: rotate(360deg);
}
}
Make sure to import styles/globals.css
in your _app.js
or _app.tsx
file:
import '../styles/globals.css'
function MyApp({ Component, pageProps }) {
return <Component {...pageProps} />
}
export default MyApp
Step 6: Use the plugin in a component
Create a new component called PageList.js
:
import { useState, useEffect } from 'react'
const MOCK_DATA = [
{ id: 1, title: 'Page 1' },
{ id: 2, title: 'Page 2' },
{ id: 3, title: 'Page 3' },
]
const PageList = () => {
const [pages, setPages] = useState([])
const [loading, setLoading] = useState(true)
useEffect(() => {
fetchPages()
}, [])
const fetchPages = async () => {
// mock api call to and to show spinner for two seconds
const data = await new Promise((resolve) => {
setTimeout(() => {
resolve(MOCK_DATA)
}, 2000)
})
setPages(data)
setLoading(false)
}
return (
<div>
{loading ? (
<div className="loading-indicator"></div>
) : (
<ul>
{pages.map((page) => (
<li key={page.id}>{page.title}</li>
))}
</ul>
)}
</div>
)
}
export default PageList
In this component, we fetch a list of pages and display them. While the pages are being fetched, we show a loading spinner using the .loading-indicator
utility class from our plugin.
Step 7: Add the PageList component to your application
Finally, let’s use the PageList
component in the index.js
file:
import Head from 'next/head'
import PageList from '../components/PageList'
export default function Home() {
return (
<div>
<Head>
<title>My Next App</title>
<link rel="icon" href="/favicon.ico" />
</Head>
<main>
<h1 className="text-2xl font-bold">List of Pages</h1>
<PageList />
</main>
</div>
)
}
Now, run the development server:
npm run dev
Open your browser and navigate to http://localhost:3000
. You should see the loading spinner while the list of pages is being fetched, and the list of pages once the data is loaded.
That’s it! You have successfully created a Next.js project with Tailwind CSS and implemented a simple plugin to style loading elements.
- Tailwind CSS Documentation: The official Tailwind CSS documentation covers the framework in depth and provides a wealth of information on its features, usage, and customization options. It’s a great starting point to learn more about Tailwind CSS and its ecosystem:
- Next.js Documentation: The official Next.js documentation is an excellent resource for learning about the framework and its features. It includes guides, examples, and best practices for building React applications with Next.js:
- Tailwind CSS Plugin Creation Guide: This guide from the official documentation provides an overview of creating custom plugins in Tailwind CSS. It covers the basics of plugin creation, the plugin API, and offers examples:
- Tailwind CSS GitHub Repository: The official GitHub repository for Tailwind CSS contains the source code, examples, and a wealth of community-contributed plugins and resources:
- Next.js Examples: The Next.js GitHub repository provides many example projects that showcase how to integrate various technologies and libraries with Next.js, including Tailwind CSS:
- Tailwind CSS Community Plugins: A list of community-contributed plugins for Tailwind CSS. Exploring these plugins can give you an idea of what’s possible and inspire you to create your own plugins:
- Tailwind CSS Labs: The official GitHub organization for experimental projects and ideas related to Tailwind CSS. You can find interesting projects and plugins that may eventually become part of the core framework:
-
How to Start Learning Python: A Beginner’s Guide
Introduction: Python has emerged as one of the most popular programming languages, renowned for its simplicity and versatility. Whether you’re eyeing a career in software development, or data science, or just looking to expand your technical skills, learning Python is a valuable investment. This guide offers a step-by-step approach to kickstart your Python learning journey. […]
-
Embracing Japan’s Autumn: A Kaleidoscope of Tradition, Beauty, and Gastronomy
Explore Japan’s autumn – from lovely foliage and hot springs to harvest festivals and food events, making your visit an unforgettable journey into tradition, beauty, and gastronomy.
-
Top Fall Travel Destinations in Europe
# _Top Fall Travel Destinations in Europe:Explore the Autumn Charm_ Fall is a magical season, when the world explodes in a kaleidoscope of colors. From fiery reds to vibrant oranges, the leaves turn delightful shades that paint a beautiful picture against the cool blue sky. While many associate fall with pumpkin spice lattes and cozy […]
2 Comments
Creating own plugins for sure have its benefits. It is easy to implement custom classes that can extend the core utility classes that Tailwind offers. But I’m skeptical that this would scale well for medium to large applications, since we now have to look into another file and probably this wouldn’t play well in a team environment.
[…] How to Master Tailwind CSS Plugin Development in Your Next.js Projects: A Comprehensive Guide […]