Elevating Your JavaScript Skills: Introducing 5 Operators You Haven’t Heard Of
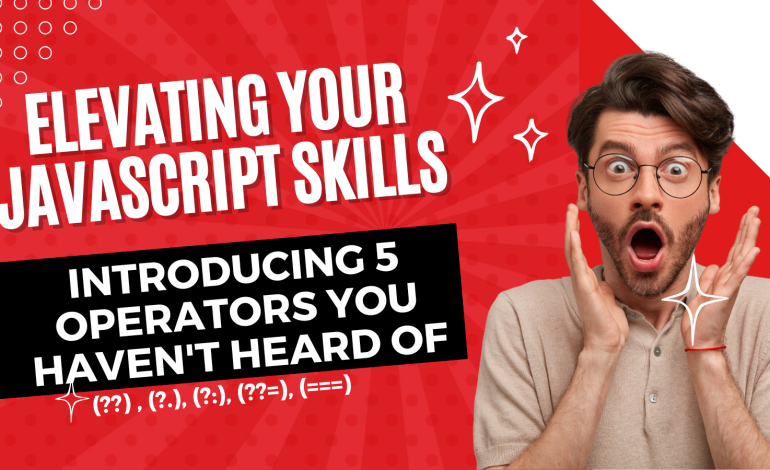
As a developer, I’ve often noticed that not everyone on a team is familiar with the latest features of the language or the newest tools available. This can be particularly challenging when working on a team where junior and mid-level developers are still learning the ropes. In some cases, I’ve encountered situations where these developers aren’t familiar with newer features like the optional chaining operator, nullish coalescing operator, conditional operator, nullish assignment operator, and nullish equality operator. By introducing these operators to the team, you can help them become better developers, Elevating Your JavaScript Skills by writting more efficient code, and stay up-to-date with the latest developments in the language. It’s always important to share knowledge and help others grow in their skills, and introducing new features and tools can be a great way to do that.
Nullish coalescing operator (??)
const myValue = null;
const result = myValue ?? 'default value';
console.log(result); // Output: 'default value'
The nullish coalescing operator (??
) returns the right-hand operand if the left-hand operand is null or undefined, otherwise it returns the left-hand operand. In the example above, myValue
is null, so the result of the expression is the default value of 'default value'
.
Optional chaining operator (?.)
const myObject = {
nested: {
property: 'value'
}
};
const result = myObject?.nested?.property;
console.log(result); // Output: 'value'
The optional chaining operator (?.
) allows you to safely access nested properties or methods of an object without worrying about whether intermediate properties are null or undefined. In the example above, the ?.
operator is used to safely access the property
property of the nested
object. If either myObject
or myObject.nested
were null or undefined, the result would be undefined.
Conditional operator (?:)
const myValue = null;
const result = myValue ? 'not null' : 'null';
console.log(result); // Output: 'null'
The conditional operator (?:
) is similar to an if-else statement, but can be used in a more concise way. In the example above, if myValue
is null or undefined, the result is 'null'
, otherwise the result is 'not null'
.
Nullish assignment operator (??=)
let myVariable = null;
myVariable ??= 'default value';
console.log(myVariable); // Output: 'default value'
The nullish assignment operator (??=
) allows you to assign a value to a variable only if the variable is currently null or undefined. In the example above, myVariable
is null, so it is assigned the value of 'default value'
.
The Strict Equality Comparison(===)
console.log(null === undefined); // Output: false
console.log(null ?? undefined); // Output: undefined
The strict equality operator (===
), but considers null and undefined to be equal to each other (but not equal to any other value). In the first example above, null
and undefined
are not considered equal, so the result is false
. In the second example, the nullish coalescing operator (??
) returns undefined
, which is not equal to null
, so the result is undefined
.
Summary
Elevating Your JavaScript Skills with knowing mentioned operator in your next project. JavaScript has introduced new operators like the optional chaining operator, nullish coalescing operator, conditional operator, nullish assignment operator, and nullish equality operator. These operators can help developers write more efficient, concise, and readable code. However, not all developers may be aware of these new features, particularly those who are newer to the language. By introducing these operators to the team, you can help them become better developers and stay up-to-date with the latest developments in the language. Sharing knowledge and helping others grow in their skills is an important part of being a developer.
Useful Links
- Mozilla Developer Network (MDN): JavaScript Nullish Coalescing Operator: https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Operators/Nullish_coalescing_operator
- JavaScript.info: Optional Chaining ‘?.’: https://javascript.info/optional-chaining
- LogRocket: A Guide to the JavaScript Conditional (Ternary) Operator: https://blog.logrocket.com/a-guide-to-the-javascript-conditional-ternary-operator/
- JavaScript Nullish Assignment: https://javascript.plainenglish.io/javascript-nullish-assignment-4f7e171b8089
- SitePoint: A Comprehensive Guide to JavaScript’s Conditional (Ternary) Operator: https://www.sitepoint.com/javascript-ternary-operator/
- A Turning Point in the War on Drugs: The Arrests of Ismael ‘El Mayo’ Zambada and Joaquín Guzmán López
- A Turning Point in the War on Drugs: The Arrests of Ismael ‘El Mayo’ Zambada and Joaquín Guzmán López
- NFL Training Camp: Najee Harris, Jack Conklin Among Veterans Who Face Increased Competition
- Dylan Cease Delivers a Historic No-Hitter: A Triumph for the Padres
- NFL Training Camp: Najee Harris, Jack Conklin Among Veterans Facing Change
1 Comment
??= was new to me