Best Practices & Clean Code Tips for JavaScript Variables
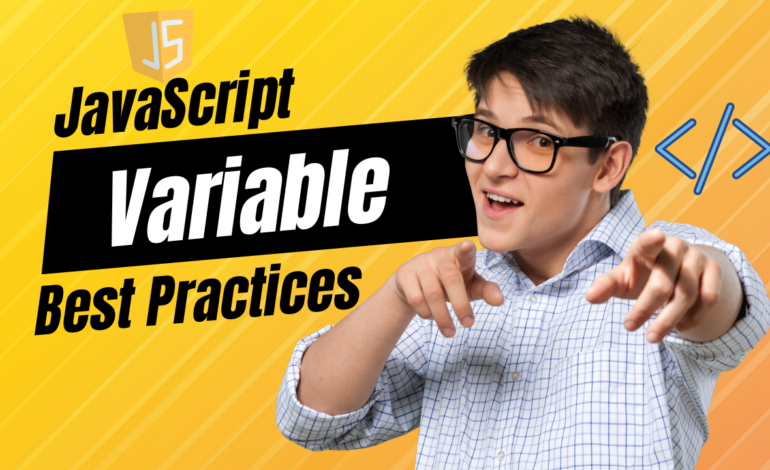
As a JavaScript developer, you will find that properly defining and managing your variables is very important not only for the performance of your code but also for the readability and maintainability of your codebase.
This article will cover the best practices and clean code tips for variables in JavaScript. It will cover everything from variable naming conventions to scope management and the use of variable types.
Table of Contents
- Understand Variable Naming Conventions
- Use let and const instead of var
- Be Crystal Clear with Your Variable Declarations
- Declare all Your Variables at the Top of Your Scope
- Avoid Global Variables
- Make Sure to Always Initialize Your Variables
- Use the Correct Variable Type for the Task at Hand
- Avoid Using Magic Values
- Don’t Ignore Linting Tools
If you’re ready to take your variable management to the next level, let’s dive into each of these best practices and clean code tips in more detail.
Understand Variable Naming Conventions
Having a consistent naming convention for your variables will make your code more readable and easier to maintain. The convention for naming variables in JavaScript is camelCase. CamelCase refers to starting the first word with lowercase and then capitalizing the first letter of each following word.
For example, if you want to declare a variable to store the user’s name, you would write it like this:
let userName = "John Doe";
Use let and const instead of var
In old versions of JavaScript, it was common to use the keyword var
to declare variables. However, the introduction of let
and const
in ECMAScript 6 (ES6) has made it easier to manage your variables and avoid variable scope issues.
Use let
when you want to declare a variable that will be reassigned later in your code. Use const
when you want to declare a variable that shouldn’t be reassigned.
let counter = 0; // can be reassigned
const BASE_URL = "https://example.com"; // cannot be reassigned
Be Crystal Clear with Your Variable Declarations
Be explicit about the type of the variable when you declare it. This will make your code easier to understand and maintain. For example, if you’re declaring a variable that will store a number, prefix it with num
.
let numStudents = 25; // number
let strName = "John"; // string
let arrNumbers = [1, 2, 3]; // array
let objPerson = {name: "John Doe", age: 30}; // object
Declare all Your Variables at the Top of Your Scope
JavaScript has hoisting, which is a mechanism that moves variable and function declarations to the top of their respective scopes. However, this can lead to unexpected behavior and bugs. To avoid this, declare all your variables at the top of their respective scope.
function calculate() {
let num1 = 5;
let num2 = 10;
//...some code
let sum = num1 + num2;
return sum;
}
Avoid Global Variables
Global variables are accessible from any code in your application. This makes it easy to accidentally change them, leading to subtle bugs or unintended consequences. To avoid this, try to minimize the use of global variables by keeping your variables scoped to the functions or modules that need them.
Make Sure to Always Initialize Your Variables
Always initialize your variables when you declare them. This will help you avoid undefined or null errors later on in your code.
let num = 0; // initialized to 0
let str = ""; // initialized to an empty string
Use the Correct Variable Type for the Task at Hand
A magic value is a hard-coded value that has no clear meaning or context. Avoid using magic values in your code by creating constants or enums.
const MAX_ATTEMPTS = 3;
Don’t Ignore Linting Tools
Linting tools can help you catch errors and maintain a consistent coding style across your project. Use one of the many available linting tools, such as ESLint or JSHint, and configure it to your specific needs.
Conclusion
By following these best practices and clean code tips for variables in JavaScript, you can write more maintainable and expressive code. Your fellow developers will thank you, and your future self will appreciate the time you invested in writing clean and readable code. I you want to learn more about best practices and clean code tips take a look at this amazing repository about clean code in JavaScript. Also in case you wanted to learn Next.js check out my previous article on Performance Optimization in Next.js.