10 Must-Know JavaScript Questions for Frontend Technical Interviews
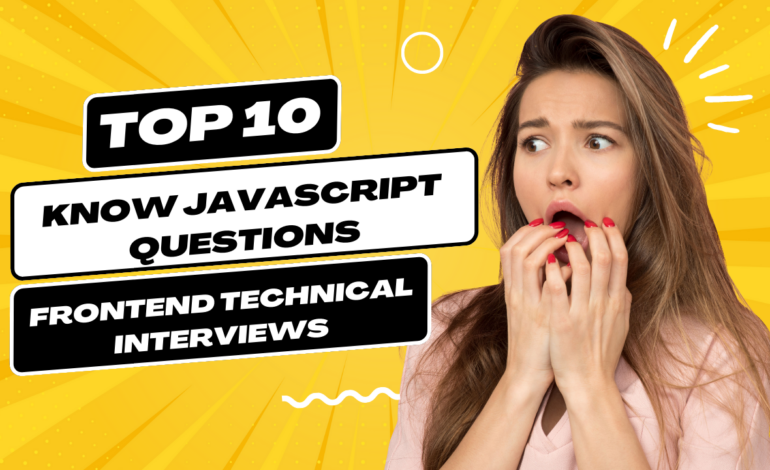
Recently, I landed a frontend developer job after going through a series of technical interviews. One of the key challenges I faced was mastering JavaScript, a fundamental language used extensively in frontend development. To help others facing the same challenge, I have compiled a list of 10 Must-Know JavaScript Questions that you are likely to encounter in a technical interview.
1. What is closure in JavaScript and how is it used?
The closure is a powerful feature in JavaScript that allows a function to access its outer lexical scope even after it has returned. In other words, a closure is formed when an inner function is returned from an outer function and the inner function has access to the outer function’s variables, parameters, and functions.
Here is an example of a closure:
function outerFunction() {
let outerVariable = 'Hello';
function innerFunction() {
console.log(outerVariable);
}
return innerFunction;
}
let innerFunc = outerFunction();
innerFunc(); // Output: Hello
In this example, the outerVariable
is accessible to the innerFunction
even after the outerFunction
has returned. This is because innerFunction
forms a closure over the outerVariable
when it is returned from outerFunction
.
Reference: MDN Web Docs — Closures
2. What is the difference between == and === in JavaScript?
The double equals (==
) and triple equals (===
) are comparison operators in JavaScript. The main difference between them is that the double equals operator performs type coercion while the triple equals operator does not.
Type coercion is the process of converting a value from one data type to another. For example, when comparing a number to a string using the double equals operator, JavaScript will try to convert the string to a number before performing the comparison. This can sometimes lead to unexpected results.
Here are some examples:
console.log(5 == '5'); // Output: true
console.log(5 === '5'); // Output: false
console.log(5 == true); // Output: true
console.log(5 === true); // Output: false
In the first example, the double equals operator coerces the string '5'
to the number 5
before performing the comparison, so it returns true
. In the second example, the triple equals operator compares the values without coercion, so it returns false
. In the third example, the double equals operator coerces the boolean true
to the number 1
before performing the comparison, so it returns true
. In the fourth example, the triple equals operator compares the values without coercion, so it returns false
.
Reference: MDN Web Docs — Equality comparisons and sameness
3. How does event delegation work in JavaScript?
Event delegation is a technique in JavaScript that allows you to attach a single event listener to a parent element, instead of attaching multiple event listeners to its child elements. When an event is triggered on a child element, the event bubbles up to the parent element and can be handled by the single event listener.
Here is an example:
<ul id="list">
<li>Item 1</li>
<li>Item 2</li>
<li>Item 3</li>
</ul>
<script>
let list = document.getElementById('list');
list.addEventListener('click', function(event) {
if (event.target.tagName === 'LI') {
console.log(event.target.textContent);
}
});
</script>
In this example, we attach a single click event listener to the list
element. When a user clicks on one of the li
elements, the event bubbles up to the list
element and is handled by the event listener. We can then use the event.target
property to determine which li
element was clicked and perform the desired action.
Reference: Javascript.info— Event delegation
4. What is the difference between let and var in JavaScript?
In JavaScript, let
and var
are both used to declare variables, but they have different scoping rules. var
is function-scoped, meaning that a variable declared with var
is accessible throughout the entire function, even outside its block. let
is block-scoped, meaning that a variable declared with let
is only accessible within its block.
Here is an example:
function exampleFunction() {
if (true) {
var x = 'Hello';
let y = 'World';
}
console.log(x); // Output: Hello
console.log(y); // Uncaught ReferenceError: y is not defined
}
exampleFunction();
In this example, thevar
variablex
is accessible outside theif
block, while thelet
variabley
is not. This is becausevar
is function-scoped, sox
is declared in the function scope and accessible throughout the entire function. On the other hand,let
is block-scoped, soy
is only accessible within theif
block.
5. How do you handle asynchronous operations in JavaScript?
Asynchronous operations in JavaScript can be handled using callbacks, promises, or async/await syntax.
Callbacks are functions that are passed as arguments to other functions, and are executed when an operation completes. Callbacks are used extensively in JavaScript, but can lead to callback hell if they are nested too deeply.
Promises provide a more structured way to handle asynchronous operations by returning an object that represents the eventual completion (or failure) of an operation. Promises can be chained and handled using then()
and catch()
methods.
Async/await is a more recent addition to JavaScript that allows asynchronous operations to be handled using synchronous-style code. The async
keyword is used to declare an asynchronous function, and the await
keyword is used to pause the function until an asynchronous operation completes.
Here is an example using promises:
function fetchJSON(url) {
return fetch(url)
.then(response => {
if (!response.ok) {
throw new Error('Network response was not ok');
}
return response.json();
})
.then(data => {
console.log(data);
})
.catch(error => {
console.error('Error:', error);
});
}
fetchJSON('https://jsonplaceholder.typicode.com/todos/1');
In this example, we use the fetch()
API to retrieve data from a JSON API. We return a promise that resolves with the parsed JSON data if the response is ok, or rejects with an error if the response is not ok. We then chain a then()
method to log the data, or a catch()
method to log the error.
Reference: MDN Web Docs — Asynchronous JavaScript
6. How do you create and use classes in JavaScript?
- How do you create and use classes in JavaScript?
JavaScript classes are a more recent addition to the language, introduced in ECMAScript 2015. They provide a way to define and instantiate objects using a class syntax similar to other object-oriented languages.
To create a class, you use the class
keyword, followed by a constructor method and any other methods or properties you want to add. You can then create instances of the class using the new
keyword.
Here is an example:
class Rectangle {
constructor(height, width) {
this.height = height;
this.width = width;
}
get area() {
return this.height * this.width;
}
}
let rect = new Rectangle(5, 10);
console.log(rect.area); // Output: 50
Reference: MDN Web Docs — Classes
7. What is the difference between a callback function and a promise in JavaScript?
a callback function is passed as an argument to another function, and is executed when an operation completes. A promise, on the other hand, is an object that represents the eventual completion (or failure) of an asynchronous operation
function fetchJSON(url, callback) {
let xhr = new XMLHttpRequest();
xhr.onreadystatechange = function() {
if (xhr.readyState === 4 && xhr.status === 200) {
callback(JSON.parse(xhr.responseText));
}
};
xhr.open('GET', url);
xhr.send();
}
fetchJSON('https://jsonplaceholder.typicode.com/todos/1', function(data) {
console.log(data);
});
Reference: MDN Web Docs — Callbacks
8. How do you handle errors in JavaScript?
Errors can be handled using try-catch statements, which allow you to catch and handle exceptions thrown during the execution of a block of code. You can also throw your own errors using the throw
keyword, and handle them using try-catch statements as well.
try {
let x = 1 / 0;
if (!Number.isFinite(x)) {
throw new Error('Division by zero');
}
} catch (error) {
console.error(error);
}
9. What is the difference between function declarations and function expressions in JavaScript?
Definition: function declarations and function expressions are two ways to define functions in JavaScript
Example:
// Function declaration
function add(a, b) {
return a + b;
}
// Function expression
let multiply = function(a, b) {
return a * b;
}
Reference: MDN Web Docs — Functions
10. What is event bubbling in JavaScript?
Definition: event bubbling is a phenomenon in JavaScript where events that occur on a child element also trigger events on its parent elements, all the way up the DOM tree.
Example:
<div id="outer">
<div id="inner">
<button>Click me</button>
</div>
</div>
<script>
let outer = document.getElementById('outer');
let inner = document.getElementById('inner');
let button = document.querySelector('button');
outer.addEventListener('click', function(event) {
console.log('Outer clicked');
});
inner.addEventListener('click', function(event) {
console.log('Inner clicked');
});
button.addEventListener('click', function(event) {
console.log('Button clicked');
});
</script>
Reference:Javascript.info— Event bubbling and capturing
For each of these questions, it’s important to have a clear understanding of the concept being tested and be able to apply it in code. Practice writing code examples and experimenting with different scenarios to solidify your understanding.
In addition to understanding the concepts, it’s also important to be able to explain them clearly and concisely in an interview setting. Practice explaining these concepts to a friend or family member who is not familiar with programming, and get feedback on how understandable your explanations are.
Finally, make sure to stay up to date with the latest developments in JavaScript and web development in general. Follow blogs and websites such as MDN Web Docs, CSS-Tricks, and Smashing Magazine to stay informed about new features and best practices.
By preparing thoroughly and practicing regularly, you can feel confident and ready to tackle any technical interview for a frontend engineering position. Good luck!
1 Comment
Thank you @reactdojo for the very useful article!
One important tip for preparing for a frontend engineering interview is to familiarize yourself with common tools and frameworks used in the industry. This may include popular JavaScript libraries like React, Angular, or Vue, as well as CSS preprocessors like Sass or Less. It’s also a good idea to be comfortable with version control systems like Git and have a basic understanding of deployment and hosting options for web applications.