The Power of Pure Components: A Guide to When and How to Use Them
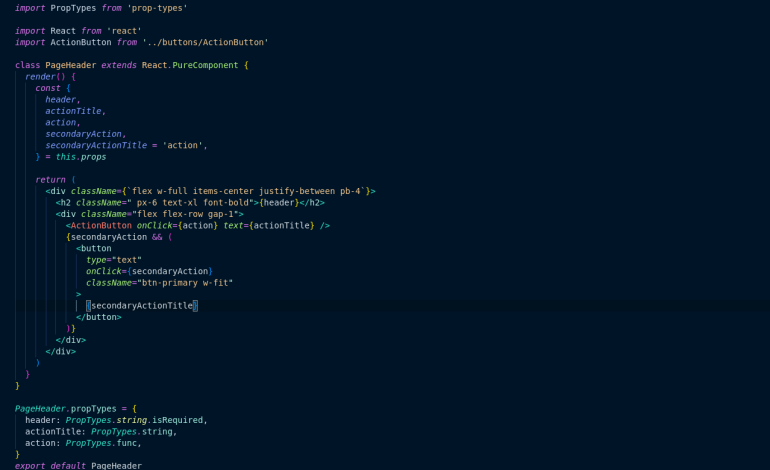
React is a popular library for building web user interfaces. One of the key concepts in React is the use of components to organize and reuse code. However, not all components are created equal. Pure components are a powerful tool in React for optimizing performance and reducing unnecessary rerenders. In this article, we’ll explore what pure components are, when to use them, and how to implement them in your React application.
What are Pure Components?
In React, a pure component is a component that only rerenders when its props or state change. This is in contrast to a regular component, which will rerender whenever its parent component rerenders, regardless of whether its props and state have changed.
Pure components achieve this by using a technique called shallow comparison. When a pure component receives new props, it will only rerender if the new props are different from the previous props. Similarly, when a pure component’s state changes, it will only rerender if the new state is different from the previous state.
When to Use Pure Components
Pure components are a great tool to use when you have a component that is expensive to render, but doesn’t need to rerender frequently. By using a pure component, you can avoid unnecessary rerenders and optimize the performance of your application.
Some examples of when to use pure components include:
- A list item component that is used multiple times on a page, but only needs to rerender when its data changes
- A form component that only needs to rerender when its input values change, not when other components on the page rerender
- A component that fetches data from an API and only needs to rerender when the data changes.
How to Implement Pure Components
Implementing a pure component in React is straightforward. You can create a pure component by extending the React.PureComponent
class instead of the regular React.Component
class. For example:
import React from 'react';
class MyPureComponent extends React.PureComponent {
render() {
return <div>{this.props.data}</div>;
}
}
In this example, MyPureComponent
is a pure component that only rerenders when the data
prop changes. It’s important to note that pure components only work with shallow comparisons. This means that if your component receives an object or array as a prop, it will only rerender if the reference to that object or array changes, not if the contents of the object or array change. If you need to perform a deep comparison, you’ll need to use a library such as lodash’s isEqual
function.
Conclusion
Pure components are a powerful tool in React for optimizing performance and reducing unnecessary rerenders. By using pure components, you can ensure that your application remains performant and responsive, even as it scales to handle larger data sets and more complex user interfaces. Remember to use pure components when you have a component that is expensive to render, but doesn’t need to rerender frequently, and to extend the React.PureComponent
class when creating your pure components.