Understanding the Difference Between Constant and Immutable Variables in Solidity
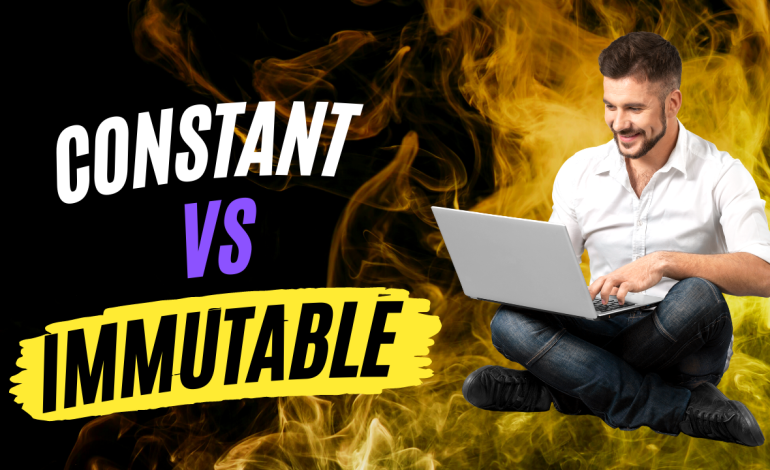
A smart contract is a self-executing computer program that automatically enforces the rules and regulations of a contract. It is designed to facilitate, verify, and enforce the negotiation or performance of a contract. Smart contracts are typically written in programming languages such as Solidity and are stored on a blockchain network.
Once deployed to a blockchain network, a smart contract is executed automatically when predefined conditions, which are written into the code, are met. Smart contracts are decentralized, meaning that they are executed on a blockchain network without the need for intermediaries or central authority. Solidity is the most popular programming language used to develop smart contracts on the Ethereum blockchain. When programming smart contracts in Solidity, it is important to understand the difference between two commonly used keywords when declaring variables: constant
and immutable
. These keywords are used to define how the value of a variable can be changed during the execution of a smart contract.
//SPDX-License-Identifier: MIT
pragma solidity ^0.8.0;
interface IERC721 {
function transferFrom (address _from, address _to, uint256 _nftId) external;
}
contract Auction{
uint256 private constant DURATION = 30 days;
IERC721 public immutable nft;
uint256 public immutable nftId;
address payable public immutable seller;
uint256 public immutable startingPrice;
uint256 public immutable startAt;
uint256 public immutable expiresAt;
uint256 public immutable discountRate;
constructor(
uint256 _startingPrice,
uint256 _discountRate,
address _nft,
uint256 _nftId
){
seller = payable(msg.sender);
startingPrice = _startingPrice;
startAt = block.timestamp;
expiresAt = block.timestamp + DURATION;
discountRate = _discountRate;
nft = IERC721(_nft);
nftId= _nftId;
}
}
Constant Variables in Solidity
A constant variable is a variable whose value can never be changed after it has been assigned a value during compilation. The value assigned to the constant variable is set at compile time and cannot be modified at runtime. This means that a constant variable is a read-only variable that can be accessed by other functions and contracts.
Constant variables are useful when you need to define values that never change, such as mathematical constants or values that do not depend on external factors. A good example of a constant variable in Solidity is the variable ‘DURATION’ in the following example smart contract:
//SPDX-License-Identifier: MIT
pragma solidity ^0.8.0;
contract Auction{
uint256 private constant DURATION = 30 days;
// ...
}
In this example, the ‘DURATION’ variable has been declared as a constant variable with a value of 30 days. Once the contract is compiled, the value of the ‘DURATION’ variable cannot be changed. Therefore, any attempt to modify its value will result in a compilation error. In Solidity, constant variables are useful for defining values that are known at compile-time and will not change during runtime. Constant variables are also used to optimize gas usage, as their value is known at compile-time and can be hard-coded into the bytecode.
Immutable Variables in Solidity
An immutable variable, on the other hand, is a variable whose value can only be set once during the constructor execution. Once an immutable variable has been assigned a value, it cannot be modified further. However, unlike a constant variable, the value of an immutable variable can be set at runtime using the constructor.
Immutable variables are useful when you need to define values that are not known until runtime, such as the address of a contract or the ID of a non-fungible token. The auction contract presented in the article is a clear example of how immutable variables can be used effectively in Solidity.
//SPDX-License-Identifier: MIT
contract Auction{
uint256 private constant DURATION = 30 days;
IERC721 public immutable nft;
uint256 public immutable nftId;
address payable public immutable seller;
uint256 public immutable startingPrice;
uint256 public immutable startAt;
uint256 public immutable expiresAt;
uint256 public immutable discountRate;
}
The nft
, nftId
, seller
, startingPrice
, startAt
, expiresAt
, and discountRate
variables are declared as immutable variables using the immutable
keyword. Immutable variables are defined at runtime, but their value cannot be changed after they have been assigned a value.
In this smart contract, the nft
, nftId
, seller
, startingPrice
, startAt
, expiresAt
, and discountRate
variables are assigned values in the constructor function. Once they have been assigned a value, their value cannot be changed. This ensures that the values of these variables cannot be tampered with during runtime.
Immutable variables are useful for defining values that need to be set at runtime but should not be changed during the execution of the contract. Immutable variables are also used to optimize gas usage, as their value is only assigned once and then read only.
Conclusion
Constant and immutable variables are important concepts to understand when writing smart contracts in Solidity. Constant variables are defined at compile-time and their value cannot be changed at runtime, while immutable variables are defined at runtime but their value cannot be changed after they have been assigned a value.