How to use AJAX in WordPress Theme and Plugin Development
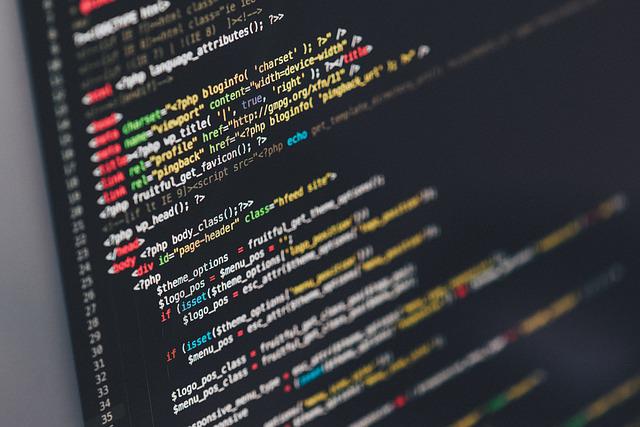
AJAX is a powerful technology that can help WordPress theme and plugin developers create dynamic, interactive websites. In this article, we’ll discuss how to use AJAX in WordPress theme and plugin development, and provide practical tips on how to get started. seo
Outline
- What is AJAX?
- Simple example of using AJAX in WordPress
- Best Practices for Using AJAX in WordPress Theme and Plugin Development
- Conclusion
What is AJAX?
AJAX is an acronym for Asynchronous JavaScript and XML. It is an advanced web technology that facilitates communication between the client and server without having to reload the page. AJAX allows developers to send and receive data from the server in the background, allowing users to access content and interact with the website without having to wait for the entire page to reload.
AJAX is based on a combination of existing web technologies, including HTML, CSS, and JavaScript. It is a powerful tool for creating dynamic, interactive websites, and is often used for tasks such as loading content, sending forms, and retrieving data from databases.
Simple example of using AJAX in WordPress
Below is an example of how AJAX can be used in WordPress to highlight unique features:
Step 1:
Create a JavaScript file to handle the AJAX call
jQuery( document ).ready( function( $ ) {
//AJAX call to get the data from the server
$.ajax( {
url: 'http://example.com/wp-admin/admin-ajax.php',
type: 'POST',
data: {
action: 'get_unique_features'
},
success: function( response ) {
//Process the response and display the data
$( '#unique-features-container' ).html( response );
}
});
});
Step 2:
Create a PHP file to handle the AJAX request
<?php
add_action( 'wp_ajax_get_unique_features', 'ajax_get_unique_features' );
add_action( 'wp_ajax_nopriv_get_unique_features', 'ajax_get_unique_features' );
function ajax_get_unique_features() {
//Get the unique features from the database
$unique_features = get_unique_features();
//Return the data as HTML
echo '<ul>';
foreach( $unique_features as $feature ) {
echo '<li>'.$feature.'</li>';
}
echo '</ul>';
wp_die();
}
Step 3:
Create a HTML file to display the data
<div id="unique-features-container"></div>
Best Practices for Using AJAX in WordPress Theme and Plugin Development
Using AJAX in WordPress theme and plugin development can offer many benefits, but it is important to keep in mind a few best practices when using AJAX. Here are some tips for using AJAX in WordPress theme and plugin development:
- Use AJAX for relevant tasks only: AJAX is a powerful tool, but it is important to use it only for tasks that require it. If a task can be completed without AJAX, it is best to avoid using it, as this can help reduce the overall server load and improve performance.
- Test your code: It is important to test your AJAX code thoroughly before deploying it on a live site. This will help ensure that your code is working correctly and is secure.
- Use proper coding conventions: It is important to use proper coding conventions when using AJAX in WordPress theme and plugin development. This will help ensure that your code is readable, maintainable, and secure.
- Use the WordPress AJAX API: WordPress provides a powerful AJAX API that can be used to simplify AJAX development in WordPress. It is important to make use of this API when possible, as it can help reduce the amount of time and effort required to develop AJAX-enabled themes and plugins.
Conclusion
AJAX is a powerful technology that can help WordPress developers create dynamic, interactive websites. In this article, we discussed how to use AJAX in WordPress theme and plugin development, and provided some tips on best practices for using AJAX in WordPress. By following these tips, you can make sure that your AJAX code is secure, readable, maintainable, and performant.
Using AJAX in WordPress theme and plugin development can offer many benefits, but it is important to remember to use it only for tasks that require it, and to test your code thoroughly before deploying it on a live site. By following these best practices, you can make sure that your AJAX code is secure, readable, maintainable, and performant.