How to Make a Custom Hook to Fetch Data from an API
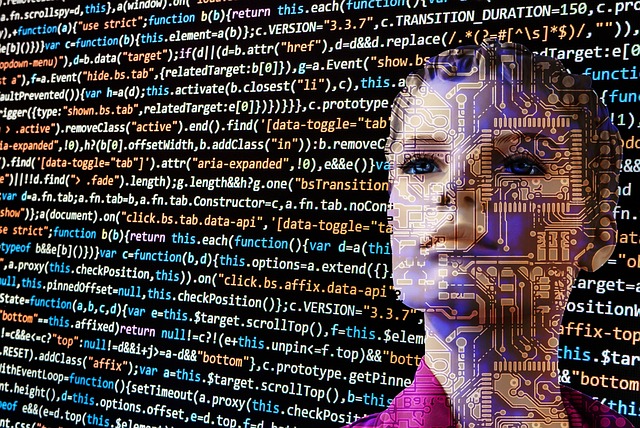
Fetching data from an API is an essential part of developing applications. It allows developers to access data from external sources quickly and easily. There are a few different methods for fetching data from an API, but one of the most popular is using a custom hook. In this article, we’ll discuss how to make a custom hook to fetch data from an API. We’ll go over what a custom hook is, how to create one, and how to use it in your application.
Outline
- What is a Custom Hook?
- How to Create a Custom Hook
- Using the Custom Hook to Fetch Data from an API
- Conclusion
What is a Custom Hook?
A custom hook is a function that allows developers to reuse logic and state between different components in a React application. This is helpful when dealing with data that needs to be accessed across multiple components. Custom hooks can also be used to fetch data from an API and store it in the local state of a component.
How to Create a Custom Hook
Creating a custom hook to fetch data from an API is actually quite simple. The first step is to create a new file in your project directory and name it something relevant to the hook you’re creating. For example, if you’re creating a hook to fetch data from the GitHub API, you might name the file “useGitHubAPI.js”.
Next, you’ll need to create a function that takes in two parameters: a URL and an optional set of options. The URL is the API endpoint you want to call and the options are the HTTP headers and query parameters you want to pass to the API.
Inside the function, you’ll need to set up a few variables. The first is a variable called “data” which will be used to store the data from the API. You’ll also need to set up a “loading” variable, which will be used to indicate whether the API call is in progress. Finally, you’ll need to set up an “error” variable, which will be used to store any errors that occur when making the API call.
Next, you’ll need to use the useEffect Hook to make the API call. Inside the useEffect Hook, you’ll use the fetch API to make the call. The fetch API takes two parameters: the URL and an options object. The URL is the API endpoint you want to call and the options object contains the HTTP headers and query parameters you want to pass to the API.
Once the fetch API call is complete, you’ll need to set the “data”, “loading”, and “error” variables to the appropriate values. If the call was successful, you’ll set the “data” variable to the response data and the “loading” and “error” variables to false. If the call was unsuccessful, you’ll set the “error” variable to the error message and the “loading” and “data” variables to false.
Finally, you’ll need to return an object containing the “data”, “loading”, and “error” variables. This object will be used to store the data from the API and indicate whether the API call is in progress or if an error occurred.
Using the Custom Hook to Fetch Data from an API
Now that you’ve created the custom hook, you can use it to fetch data from an API. To do this, you’ll need to import the custom hook into the component where you want to fetch the data. Then, you’ll need to call the custom hook with the URL of the API endpoint and any optional headers or query parameters.
The custom hook will then return an object containing the data, loading, and error variables. You can then use these variables to update the state of the component and display the fetched data.
For example, if you’re fetching a list of users from the GitHub API, you would call the custom hook with the URL of the API endpoint. The custom hook would then return an object containing the fetched data, a loading variable, and an error variable. You could then use the data to update the state of the component and display the fetched data.
Conclusion
Fetching data from an API is an essential part of developing applications. Custom hooks are an excellent way to fetch data from an API and store it in the local state of a component. In this article, we discussed how to make a custom hook to fetch data from an API. We discussed what a custom hook is and how to create one. We also discussed how to use the custom hook to fetch data from an API and store it in the local state of a component.
Creating a custom hook to fetch data from an API is a great way to quickly and easily access data from external sources. With a custom hook, you don’t have to write the same code over and over again for each component you want to access the data from. Instead, you can write the code once and reuse it whenever you need to access the data.
Custom hooks are a powerful tool for developers and can make the process of fetching data from an API much easier and more efficient.