Advanced Techniques for Efficient Data Serialization in JavasScript JSON.stringify()
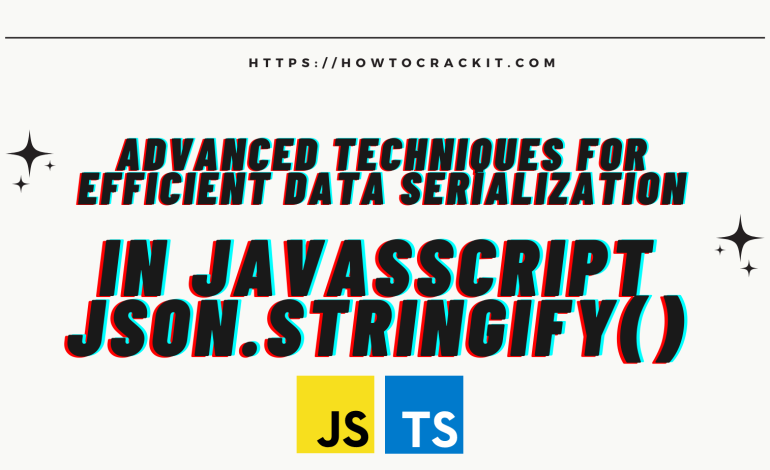
Data serialization is an essential process for transmitting and storing data in a format that can be easily interpreted by other systems. When it comes to serialization in JavaScript, JSON.stringify() is the most popular method for converting JavaScript objects into JSON format. However, when working with large or complex objects, the performance of JSON.stringify() can suffer, resulting in slow and inefficient serialization. In this article, we’ll explore some Techniques for Efficient Data Serialization for using JSON.stringify() to efficiently serialize large or complex data structures in JavaScript.
Introduction to JSON.stringify()
JSON.stringify() is a built-in method in JavaScript that allows you to convert a JavaScript object into a JSON string. This is useful for transmitting data over a network, storing data in a file, or passing data between different systems that speak different languages. By default, JSON.stringify() serializes all properties of an object, including functions, and undefined values.
Here’s an example of how to use JSON.stringify() to serialize a simple JavaScript object:
const person = {
name: "Alice",
age: 30,
isStudent: false
};
const jsonStr = JSON.stringify(person);
console.log(jsonStr);
The output will be: {"name":"Alice","age":30,"isStudent":false}
Case 1: Removing Properties by List of Keys
In some cases, you may want to remove specific properties from an object before serializing it. For example, you may have sensitive information that you don’t want to include in the serialized data.
To remove specific properties from an object, you can pass an array of property names to the second argument of JSON.stringify(). This will exclude the specified properties from the serialized data.
Here’s an example:
const person = {
name: "Alice",
age: 30,
isStudent: false,
password: "secret123"
};
const keysToExclude = ["password"];
const jsonStr = JSON.stringify(person, keysToExclude);
console.log(jsonStr);
The output will be: {"name":"Alice","age":30,"isStudent":false}
In this example, we define an object person
with a property named “password” that we want to exclude from serialization. We pass an array of property names keysToExclude
to the second argument of JSON.stringify() to exclude the “password” property from serialization.
Case 2: Manipulating Data with a Function
Another advanced technique for efficient serialization is to pass a function as the second argument to JSON.stringify(). This function can be used to manipulate the data being serialized and modify the output.
For example, you can use this technique to convert all properties with a certain name to a different format or to exclude certain values from serialization.
Here’s an example:
const person = {
name: "Alice",
age: 30,
isStudent: false,
sensitiveData: {
password: "secret123",
creditCard: "1234567890"
}
};
const jsonStr = JSON.stringify(person, (key, value) => {
if (key === "password" || key === "creditCard") {
return undefined;
} else if (key === "isStudent") {
return value ? "Yes" : "No";
} else {
return value;
}
});
console.log(jsonStr);
The output will be: {"name":"Alice","age":30,"isStudent":"No"}
In this example, we define an object person
with a property named “sensitiveData” that we want to exclude from serialization, and we also modify the output of the “isStudent” property by returning “Yes” or “No” instead of a boolean value.
To achieve this, we pass a function as the second argument to JSON.stringify(). The function takes two arguments: the property key and the value of the property. We check if the property key is “password” or “creditCard” and return undefined to exclude these properties from serialization. We also check if the property key is “isStudent” and return “Yes” or “No” instead of the boolean value.
Summary
In this article, we explored some advanced techniques for using JSON.stringify() to efficiently serialize large or complex data structures in JavaScript. We learned how to exclude specific properties from serialization by passing an array of property names to the second argument of JSON.stringify(). We also learned how to pass a function as the second argument to manipulate the data being serialized and modify the output.
By using these advanced techniques, you can optimize the performance of JSON.stringify() and efficiently serialize large or complex data structures in JavaScript.
References
-
A Turning Point in the War on Drugs: The Arrests of Ismael ‘El Mayo’ Zambada and Joaquín Guzmán López
In a decisive blow against one of the world’s most notorious drug trafficking organizations, U.S. Attorney General Merrick B. Garland recently announced the arrest of Ismael “El Mayo” Zambada and Joaquín Guzmán López, two high-ranking figures of the Sinaloa Cartel.
-
A Turning Point in the War on Drugs: The Arrests of Ismael ‘El Mayo’ Zambada and Joaquín Guzmán López
# A Turning Point in the War on Drugs: The Arrests of Ismael ‘El Mayo’ Zambada and Joaquín Guzmán López ## Introduction In a decisive blow against one of the world’s most notorious drug trafficking organizations, U.S. Attorney General Merrick B. Garland recently announced the arrest of Ismael “El Mayo” Zambada and Joaquín Guzmán López, […]
-
NFL Training Camp: Najee Harris, Jack Conklin Among Veterans Who Face Increased Competition
NFL Training Camp: Najee Harris, Jack Conklin Among Veterans Who Face Increased Competition The excitement of NFL training camps is palpable as teams gear up for the new season. With every passing year, the stakes become higher for veteran players, especially those who a younger wave of talent is eyeing for their positions. In this […]